Till now we have learnt about how to make a GET Request using Rest-Assured. We have also learnt how to read different components of a HTTP Response (Headers, Body and Status). If you have not already read previous tutorials, please take a look at the list of tutorials here:
POST Request using Rest Assured
During this tutorial, we will learn about
- Basics of Post Verb/Method/Request
- POST Request using Rest Assured
- Creating JSON data using Simple JSON library
- Sending JSON content in the body of Request
- Validating the Response.
Now let us make a POST request using Rest-Assured and before that, we need to understand the basics of POST Request.
What is POST verb of HTTP protocol?
HTTP POST Verb often called POST Method is used to send data to the Server. Common places where you can find a POST request is when you submit Form Data (HTML forms) on a web page. For e.g. submission of the registration form of Gmail. POST request usually result in changes on the Server like the addition of new data or maybe updated to existing data.
The data that is sent to the server in a POST request is sent in the body of the HTTP request. The type of body, XML, JSON or some other format is defined by the Content-Type header. If a POST request contains JSON data then the Content-Type header will have a value of application/json. Similarly, for a POST request containing XML the Content-Type header value will be application/xml.
Let us understand testing a POST web service using a live example. We will take a look at a registration web service. Web service details are
Endpoint | http://restapi.demoqa.com/customer/register |
---|---|
HTTP method type: | POST |
Body: | {"FirstName" : "value""LastName" : "value","UserName" : "value","Password" : "value","Email" : "Value"} |
Success Response: | {"SuccessCode": "OPERATION_SUCCESS", "Message": "Operation completed successfully" } |
Failure Response: | {"FaultId": "User already exists","fault": "FAULT_USER_ALREADY_EXISTS" } |
How to make a POST Request using Rest Assured?
In order to create JSON objects in the code, we will add a Simple JSON library in the classpath. You can download Simple JSON from the maven using the following URL: https://mvnrepository.com/artifact/com.googlecode.json-simple/json-simple. Then add the downloaded Jars to the classpath.
Let us begin step by step with the code
Step 1: Create a Request pointing to the Service Endpoint
RestAssured.baseURI ="https://restapi.demoqa.com/customer";
RequestSpecification request = RestAssured.given();
This code is self-explanatory. If you are not able to understand it you have missed the earlier tutorials in this series, please go through them first.
Step 2: Create a JSON request which contains all the fields
// JSONObject is a class that represents a Simple JSON.
// We can add Key - Value pairs using the put method
JSONObject requestParams = new JSONObject();
requestParams.put("FirstName", "Virender");
requestParams.put("LastName", "Singh");
requestParams.put("UserName", "simpleuser001");
requestParams.put("Password", "password1");
requestParams.put("Email", "someuser@gmail.com");
JSONObject is a class that is present in org.json.simple package. This class is a programmatic representation of a JSON string. Take a look at the Request JSON above for our test web service, you will notice that there are multiple nodes in the JSON. Each node can be added using the JSONObject.put(String, String) method. Once you have added all the nodes you can get the String representation of JSONObject by calling JSONObject.toJSONString() method.
Step 3: Add JSON body in the request and send the Request
// Add a header stating the Request body is a JSON
request.header("Content-Type", "application/json");
// Add the Json to the body of the request
request.body(requestParams.toJSONString());
// Post the request and check the response
Response response = request.post("/register");
This web service accepts a JSON body. By this step, we have created our JSON body that needs to be sent. In this step, we will simply add the JSON String to the body of the HTTP Request and make sure that the Content-Type header field has a value of application/json.
You can put the JSON string in the body using the method called RequestSpecification.body(JsonString). This method lets you updated the content of HTTP Request Body. However, if you call this method multiple times the body will be updated to the latest JSON String.
Step 4: Validate the Response
Once we get the response back, all we have to do is validate parts of the response. Let us validate Success Code text in the Response, we will be using JsonPath. More on JsonPath can be found here.
int statusCode = response.getStatusCode();
Assert.assertEquals(statusCode, "201");
String successCode = response.jsonPath().get("SuccessCode");
Assert.assertEquals( "Correct Success code was returned", successCode, "OPERATION_SUCCESS");
Now that we have sent the Request and received a Response, let us validate Status Code and Response Body content. This is similar to the validation techniques that we discussed in previous tutorials. I would strongly suggest that you read those tutorials. The above code does the validation, it is self-explanatory.
Complete code
Here is the complete code for the above example
@Test
public void RegistrationSuccessful()
{
RestAssured.baseURI ="https://restapi.demoqa.com/customer";
RequestSpecification request = RestAssured.given();
JSONObject requestParams = new JSONObject();
requestParams.put("FirstName", "Virender"); // Cast
requestParams.put("LastName", "Singh");
requestParams.put("UserName", "sdimpleuser2dd2011");
requestParams.put("Password", "password1");
requestParams.put("Email", "sample2ee26d9@gmail.com");
request.body(requestParams.toJSONString());
Response response = request.post("/register");
int statusCode = response.getStatusCode();
Assert.assertEquals(statusCode, "201");
String successCode = response.jsonPath().get("SuccessCode");
Assert.assertEquals( "Correct Success code was returned", successCode, "OPERATION_SUCCESS");
}
Changing the HTTP Verb on a POST Request
One of the key aspect of Web service testing is to verify negative scenarios on the Endpoint. There could be many negative scenarios, some of them are
- Sending incomplete POST Data
- Sending JSON data with incorrect syntax
- Sending incorrect Verb in the Request.
Let us see what the impact will be if we send a GET request to an Endpoint that expects POST. Below code tries to do that, we will just print out the Response status code and the Response body to see if we get any error.
public void RegistrationSuccessful()
{
RestAssured.baseURI ="https://restapi.demoqa.com/customer";
RequestSpecification request = RestAssured.given();
JSONObject requestParams = new JSONObject();
requestParams.put("FirstName", "Virender"); // Cast
requestParams.put("LastName", "Singh");
requestParams.put("UserName", "sdimpleuser2dd2011");
requestParams.put("Password", "password1");
requestParams.put("Email", "sample2ee26d9@gmail.com");
request.body(requestParams.toJSONString());
Response response = request.get("/register");
int statusCode = response.getStatusCode();
System.out.println("The status code recieved: " + statusCode);
System.out.println("Response body: " + response.body().asString());
}
If you run this test following output is generated. In the output we can clearly see that the Response body tells us about the incorrect usage of HTTP Verb. The HTTP verb is also known as the Method types, given we actually make a remote method call and specify the type of the method call.
Try out further scenarios your self on the registration API that is described in this tutorial.
Also, read the next tutorial where we will use the Deserialization technique to convert the Response body into a Class instance.
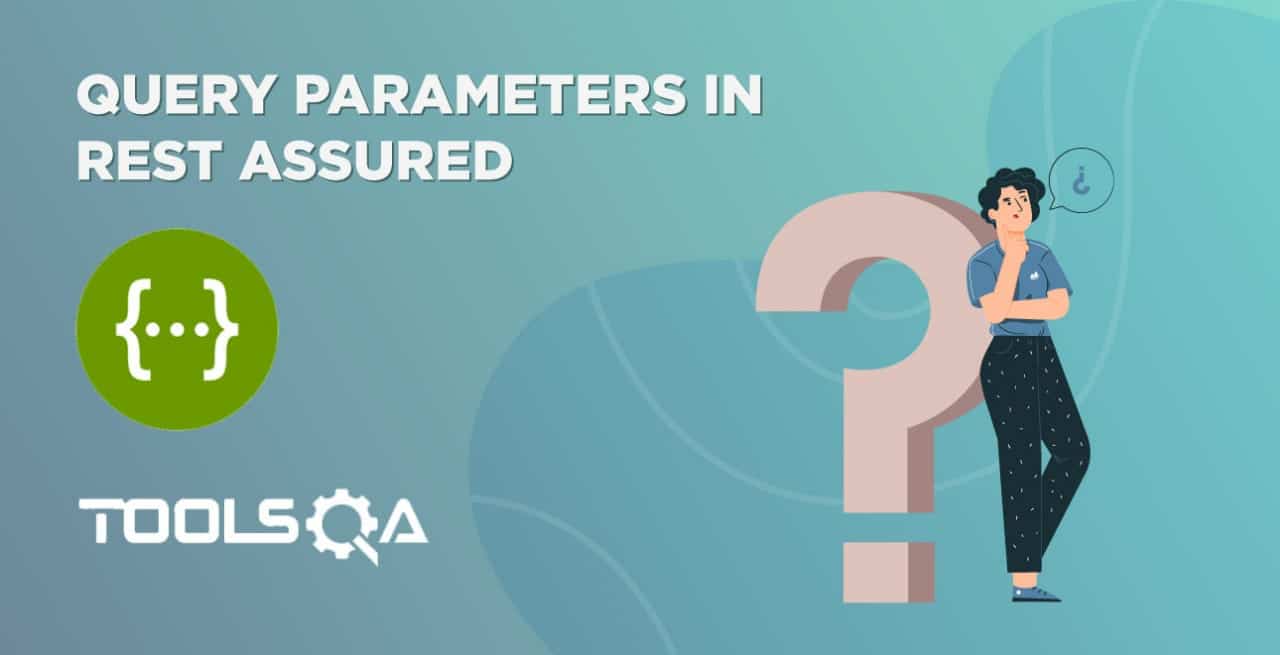
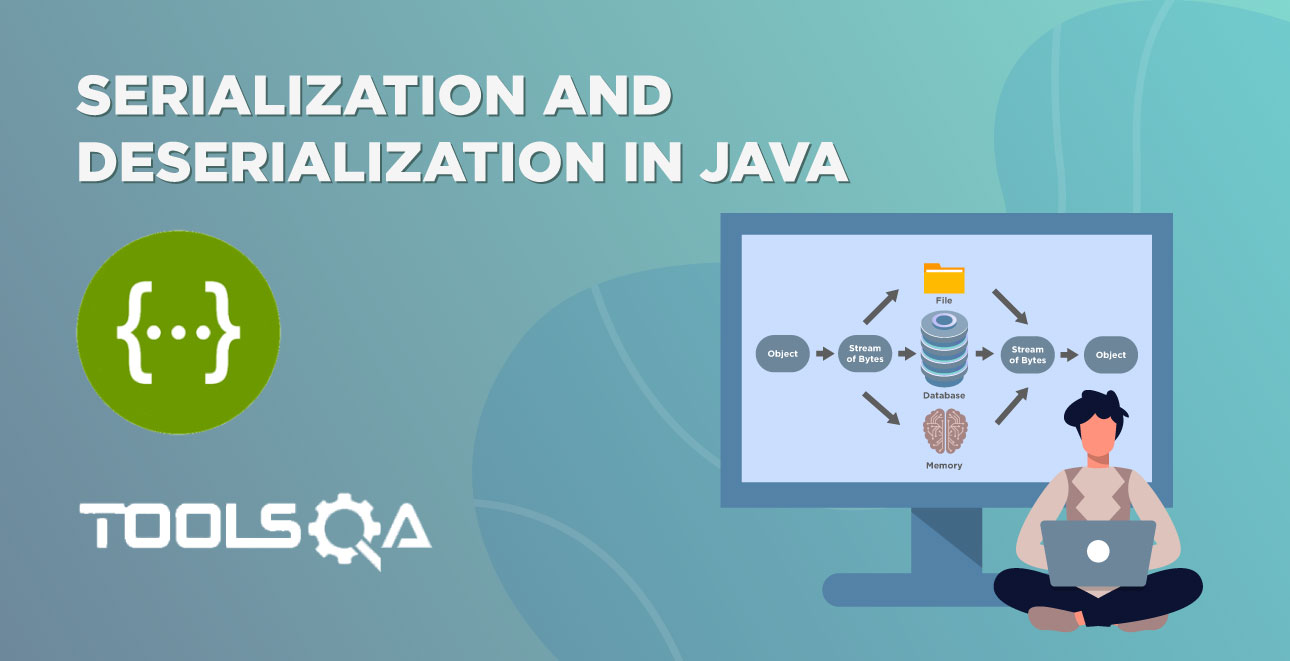