Till now, we have learned about how to make a POST Request using Rest-Assured. In addition to this, we also learned to read different components of an HTTP Response (Headers, Body, and Status). It is advisable to read the previous tutorials. In case you haven't read them yet, please take a look at the list of tutorials here:
Rest API testing using Rest-Assured
In continuation with the previous article on Authentication and Authorization in REST Web Services, we will understand the below things in this article:
- Basics of PUT Verb/Method/Request
- PUT Vs. POST Request
- Appropriate status codes obtained for PUT and POST requests
- REST API example
- How to make a PUT Request using Rest Assured
- Creating JSON data using Simple JSON library
- Sending JSON content in the body of Request
- Validating the Response
What is PUT Request?
The HTTP PUT request method creates a new resource or substitutes a representation of the target resource with the request payload.
According to the official HTTP RFC specifies PUT to be:
- A PUT method puts a file or resource at a specific URI, and precisely at that URI.
- If a file or a resource already exists at that URI, PUT replaces that file or resource.
- If no file or resource is there, PUT creates one.
- In addition to the above, Responses to this method are not cacheable.
PUT v/s POST Request
- PUT is idempotent while POST is not.
As Wikipedia puts it,
Idempotent is the property of specific procedures in mathematics and computer science, that can be implemented multiple times without altering the result beyond the initial application. To clarify:
- Therefore, the PUT method is idempotent because no matter how many times we send the same request, the results will always be the same.
- POST is not an idempotent method. In other words, Creating a POST multiple times may result in various resources getting created on the server.
- Moreover, there is no distinction between PUT and POST if the resource already exists.
- The following example will help in conceptually clarifying the difference between PUT and POST requests.
POST /vehicle-management/vehicle: Create a new device
PUT /vehicle-management/vehicle/{id} : Updates the vehicle information identified by “id”
Appropriate status codes obtained for PUT and POST requests:
POST
- 201 and a location header pointed to the new resource
- 400 if we are not able to create an item
PUT
- 204 for OK (but no content)
- 200 for OK with Body (Updated response)
- 400 if the supplied data was invalid
REST API example:
Endpoint | http://dummy.restapiexample.com/api/v1/update/15410 |
HTTP Method Type | PUT |
Body | {"name":"Zion", "salary":"1123", "age":"23" } |
Success Response | Success Status code: 200 OK{"name":"Zion","salary":"1123","age":"23"} |
Note: The employee ID 15410 used in the example, has been previously created as a resource on the server. In addition to this, we will update the associated employee information in the PUT request.
How to make a PUT Request using Rest Assured?
As explained in the previous tutorial on a POST request, to create JSON objects, we will add Simple JSON library in the classpath in the code. Subsequently, please follow the enlisted steps under the POST request using Rest Assured and add it to the classpath.
Let us begin the code step by step.
- Step 1: Create a variable empid which we intend to update with our PUT request.
int empid = 15410;
Note: The employee ID 15410 used in the example, has been previously created as a resource on the server, and we will update the associated employee information in the PUT request.
- Step 2: Create a Request pointing to the Service Endpoint
RestAssured.baseURI
="https://dummy.restapiexample.com/api/v1";
RequestSpecification request = RestAssured.given();
- Step 3: Create a JSON request which contains all the fields which we wish to update.
// JSONObject is a class that represents a Simple JSON.
// We can add Key - Value pairs using the put method
JSONObject requestParams = new JSONObject();
requestParams.put("name", "Zion");
requestParams.put("age", 23);
requestParams.put("salary", 12000);
JSONObject class is present in org.json.simple package. There are multiple nodes of JSON. However, in this example, we are adding using the JSONObject.put(String, String) method. Here, we are updating the name, age, and salary of the employee by passing the values in Request Body.
Step 4. Send JSON content in the body of Request and pass PUT Request
// Add a header stating the Request body is a JSON
request.header("Content-Type", "application/json");
// Add the Json to the body of the request
request.body(requestParams.toJSONString());
// The actual request being passed equalizes to http://dummy.restapiexample.com/api/v1/update/15410
// Here, we capture the response for PUT request by passing the associated empID in the baseURI
Response response = request.put("/update/"+ empid);
Step 5: Validate the PUT Request response received
int statusCode = response.getStatusCode();
System.out.println(response.asString());
Assert.assertEquals(statusCode, 200);
Once we send the request, we assert the status code obtained to be equivalent to 200 OK.
Putting it all together:
import org.testng.Assert;
import org.testng.annotations.Test;
import io.restassured.RestAssured;
import io.restassured.response.Response;
import io.restassured.specification.RequestSpecification;
import net.minidev.json.JSONObject;
public void UpdateRecords(){
int empid = 15410;
RestAssured.baseURI ="https://dummy.restapiexample.com/api/v1";
RequestSpecification request = RestAssured.given();
JSONObject requestParams = new JSONObject();
requestParams.put("name", "Zion"); // Cast
requestParams.put("age", 23);
requestParams.put("salary", 12000);
request.body(requestParams.toJSONString());
Response response = request.put("/update/"+ empid);
int statusCode = response.getStatusCode();
System.out.println(response.asString());
Assert.assertEquals(statusCode, 200);
}
Summary
In conclusion, so far, we have learned about the basics of PUT Request and how it is different from a POST request. JSON data was created using a simple JSON Library. Additionally, we sent it in the content of the body of Request. Moreover, we validated the response by status code as well as the response string obtained once we send the request successfully.
In the next article, we plan to understand the DELETE request in Rest Assured.
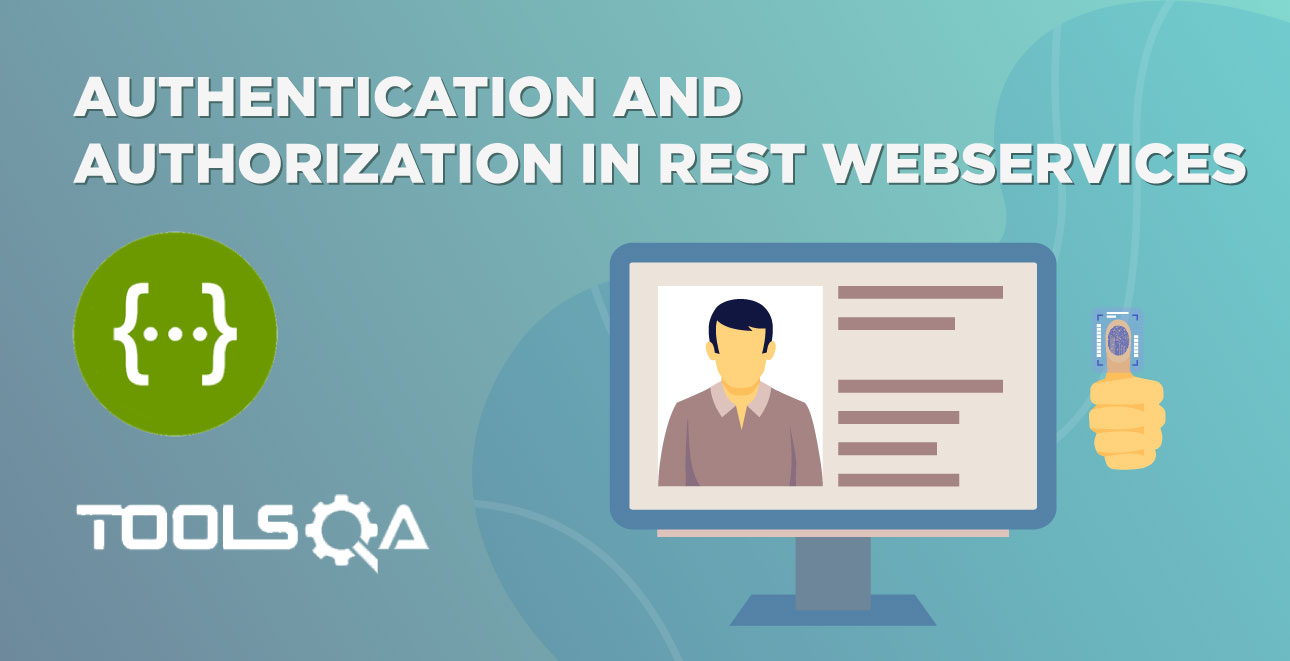
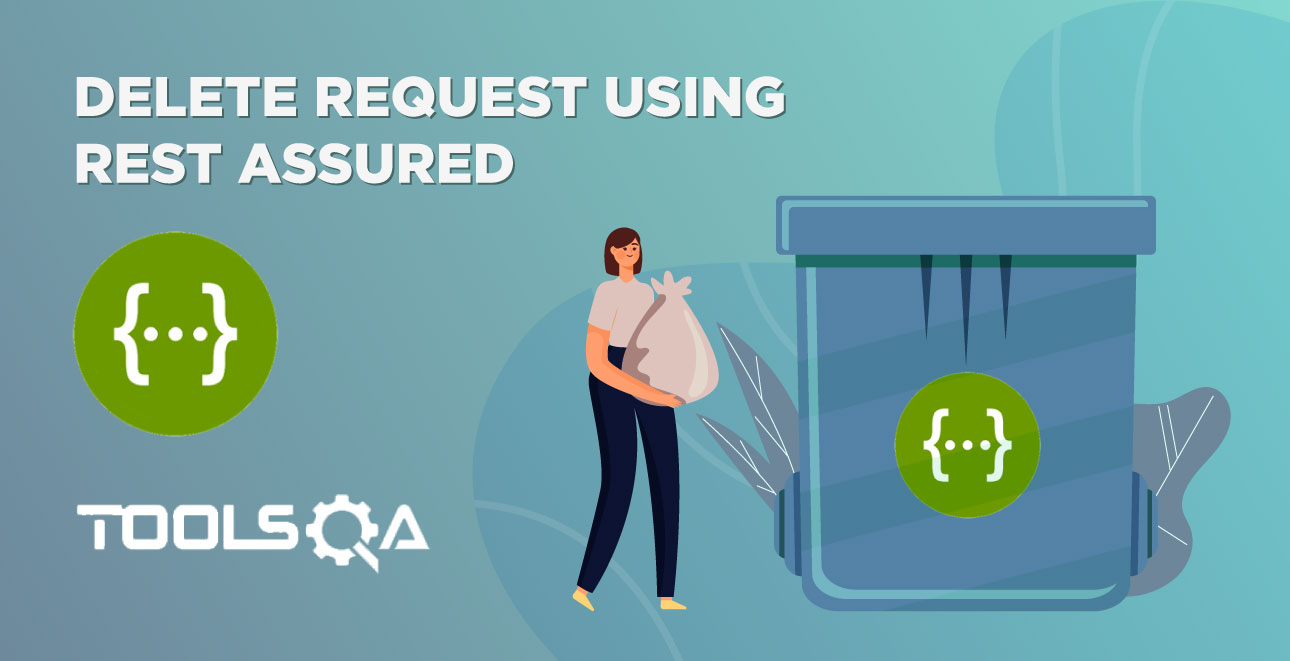