A set of parameters attached to the end of the URL is called Query Parameters. They are appended to the URL by adding '?' at the end of the URL. In addition to that, they are followed immediately with a key-value pair(Query Parameter).
In our process of learning API calls with Rest Assured, we come across an essential operation of passing Query Parameters in Rest Assured. Subsequently, let’s learn how to do it in this short post.
In this tutorial, we will try to understand the following topics, respectively:
- What is the composition of the URL?
- What do we refer to as the Query Parameter?
- Understand how to send a request with Query Parameter in Rest Assured
What is the composition of the URL?
For instance, consider a sample URL: https://www.testurl.com:9090/reviews/search?q=myntra+logistics
The table below explains the URL components available.
https:// | It is a protocol. Moreover, it talks about the application-level protocol for distributed and collaborative information systems. |
www. | It is known as a subdomain and is optional. Also, certain websites choose not to have subdomains, as well. |
testurl.com | It is a hostname or domain name. Additionally, it can be obtained from the domain registrar to name the website domain. Moreover, it often ends with .com , .net, .in, etc. |
:9090 | It is a port. It is always a number starting with the colon and placed after the host. In addition to that, it is usually not visible to the end-user. |
reviews/search | It is a path. Additionally, the directory structure of a web server determines the paths. |
?q= myntra+logistics | It is a query parameter string. Moreover, query strings are indicated with a question mark and come after the path. |
What are the Query Parameters?
In simple words, Query Parameters are a set of parameters attached to the end of the URL. Additionally, it helps to retrieve specific data and performs actions based on the inputs passed by the user. Also a ‘?’ is used immediately after the URL to append the query parameters to the URL. E.g., if you paste this URL (https://www.google.com/search?q=tiger) on the browser address bar and press enter. Consequently, google page will open with displaying Google search results for the word "tiger".
In the case of multiple parameters, we add an ‘&’ symbol in between each of the query parameters. Note that these requests are used commonly in the GET Requests only. In other words, we use the Query Parameters when an operation involves sort, pagination, or filter operation on the items.
For instance, in the below example, I am searching for the word 'tiger' on Google. As I enter the word 'tiger' in the search box, consequently, the resulting URI gets changed to:
"https://www.google.com/search?q=tiger&oq=tiger&aqs=chrome.0.69i59j0l5.1612j0j7&sourceid=chrome&ie=UTF-8"
As you can notice in the highlighted section, the query string parameters passed are:
q: | tiger |
oq: | tiger |
aqs: | chrome.0.69i59j0l5.1612j0j7 |
sourceid: | chrome |
ie: | UTF-8 |
Note: The basis of query parameters, oq, aqs, sourceid, and ie is the internal implementation of Google.
Understand how to send a request with Query Parameters in Rest Assured?
To understand how passing of Query Parameters happen in the URL in Rest Assured, consider the following open weather API service which provides current weather data for one location:
The full-service URL with the endpoint is: https://samples.openweathermap.org/data/2.5/weather?q=London,uk&appid=2b1fd2d7f77ccf1b7de9b441571b39b8
REST API Example:
Head | Head |
---|---|
Endpoint | https://samples.openweathermap.org/data/2.5/weather?q=London,uk&appid=2b1fd2d7f77ccf1b7de9b441571b39b8 |
HTTP Method Type | GET |
Query Parameters | Key: |
q: London, UK | |
appid: 2b1fd2d7f77ccf1b7de9b441571b39b8 | |
Body | We don’t need to pass the body for GET request in this example. |
Success Response
200 Status OK
{
“coord”: {
“lon”: -0.13,
“lat”: 51.51
},
“weather”: [
{
“id”: 300,
“main”: “Drizzle”,
“description”: “light intensity drizzle”,
“icon”: “09d”
}
],
“base”: “stations”,
“main”: {
“temp”: 280.32,
“pressure”: 1012,
“humidity”: 81,
“temp_min”: 279.15,
“temp_max”: 281.15
},
“visibility”: 10000,
“wind”: {
“speed”: 4.1,
“deg”: 80
},
“clouds”: {
“all”: 90
},
“dt”: 1485789600,
“sys”: {
“type”: 1,
“id”: 5091,
“message”: 0.0103,
“country”: “GB”,
“sunrise”: 1485762037,
“sunset”: 1485794875
},
“id”: 2643743,
“name”: “London”,
“cod”: 200
}
Follow the steps one by one to understand the code:
1st Step: Firstly, create a Request pointing to the Service Endpoint.
// Specify the base URL to the RESTful web service
RestAssured.baseURI ="https://samples.openweathermap.org/data/2.5/";
RequestSpecification request = RestAssured.given();
2nd Step: Secondly, send the resource details of the city, country, and the id to search the weather for in the GET request.
Response response = Request.queryParam("q","London,UK")
.queryParam("appid", "2b1fd2d7f77ccf1b7de9b441571b39b8")
.get("/weather");
3rd Step: After that, validate the GET Request response.
String jsonString = response.asString();
System.out.println(response.getStatusCode());
Assert.assertEquals(jsonString.contains("London"), true);
4th Step: Finally, the entire code would look like below.
import org.testng.Assert;
import org.testng.annotations.Test;
import io.restassured.RestAssured;
import io.restassured.response.Response;
import io.restassured.specification.RequestSpecification;
public class QUERYPARAMETERRequest {
@Test
public void queryParameter() {
RestAssured.baseURI ="https://samples.openweathermap.org/data/2.5/";
RequestSpecification request = RestAssured.given();
Response response = request.queryParam("q", "London,UK")
.queryParam("appid", "2b1fd2d7f77ccf1b7de9b441571b39b8")
.get("/weather");
String jsonString = response.asString();
System.out.println(response.getStatusCode());
Assert.assertEquals(jsonString.contains("London"), true);
}
}
Summary
To conclude, we have understood the basics of GET Request with Query Parameters in Rest Assured. We asserted the response by status code. Moreover, the response message obtained contains the expected response.
Additionally, please try and practice the GET method with Query Parameters in Rest Assured to develop more understanding of the concept.
Moreover, you can try to implement other HTTP methods that we have covered in the Rest API testing using Rest-Assured tutorial. And consequently, provide your valuable feedback.
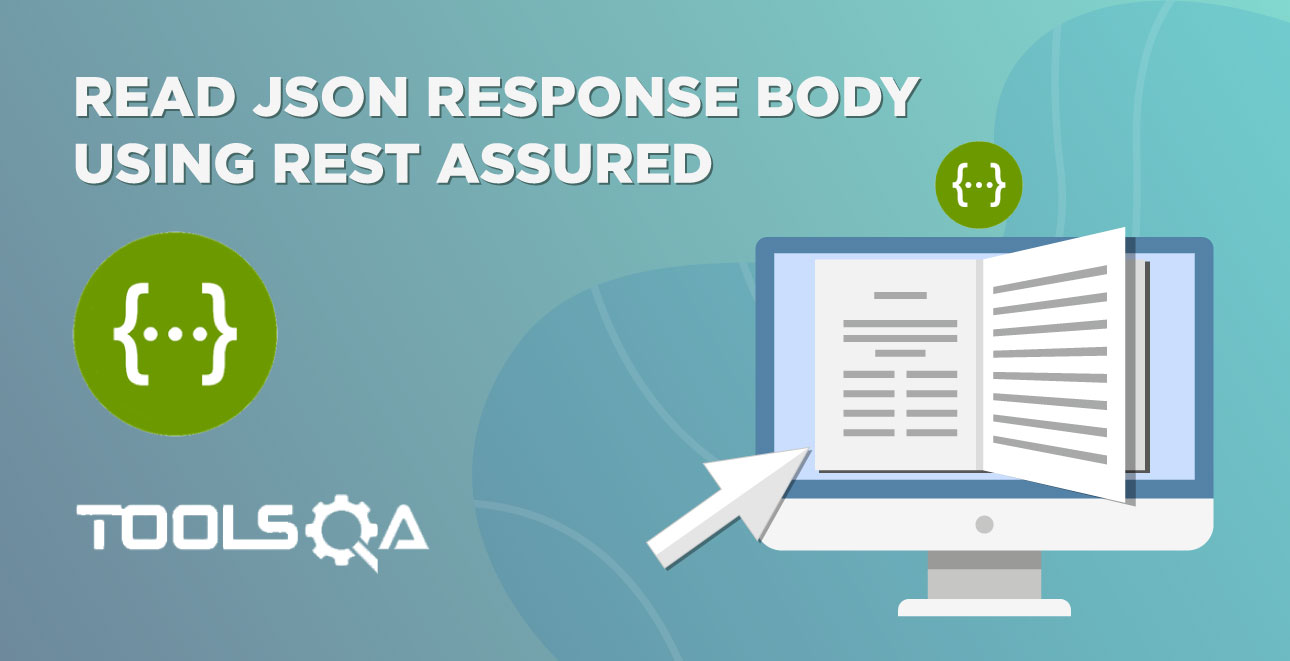
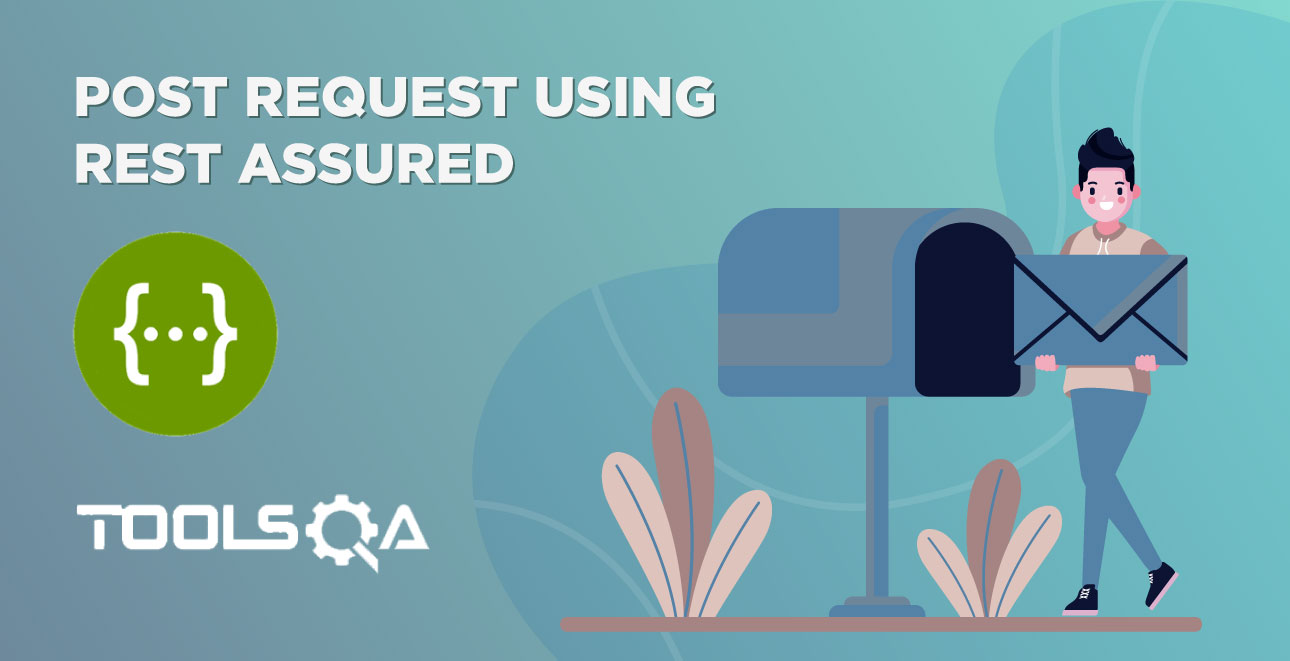