In the first chapter of Configuring Eclipse with Rest-assured, the steps to configure eclipse was shown. This chapter is all about Writing the First Rest Assured Test. This tutorial also assume that the reader must have a good knowledge of TestNG Framework. Since the Rest Assured set up is done, it is the time to write REST API Test using Rest Assured.
REST API Test using Rest Assured
This test will hit a simple Restful web service. Details of the Restful Web service are mentioned in the below table:
Endpoint | http://demoqa.com/utilities/weather/city/<City> |
HTTP method type: | GET |
Comments: | Here <City> means the city for which we are trying to retrieve the weather data. For e.g. if you want to know the weather conditions of Hyderabad, you would simple replace the <City> text with Hyderabad. The Restful resource URL for Hyderabad becomes: http://demoqa.com/utilities/weather/city/Hyderabad |
Response | { "City": "Hyderabad", "Temperature": "31.49 Degree celsius", "Humidity": "62 Percent", "Weather Description": "scattered clouds","Wind Speed": "3.6 Km per hour", "Wind Direction degree": "270 Degree" } |
Why not just try to open http://demoqa.com/utilities/weather/city/Hyderabad in the browser. Output will look like below:
In order to do the same thing using Rest-Assured, need to follow the steps below:
- Use the RestAssured class to generate a RequestSpecification for the URL: http://demoqa.com/utilities/weather/city/Hyderabad
- Specify the HTTP Method type
- Send the Request to the Server
- Get the Response back from the server
- Print the returned Response's Body
How to Write REST API Test using Rest Assured?
Below is the code to hit the above end point. Let's have a look at the code first and then at the explanation of the each line of code in the bottom.
import org.testng.annotations.Test;
import io.restassured.RestAssured;
import io.restassured.http.Method;
import io.restassured.response.Response;
import io.restassured.specification.RequestSpecification;
public class SimpleGetTest {
@Test
public void GetWeatherDetails()
{
// Specify the base URL to the RESTful web service
RestAssured.baseURI = "https://demoqa.com/utilities/weather/city";
// Get the RequestSpecification of the request that you want to sent
// to the server. The server is specified by the BaseURI that we have
// specified in the above step.
RequestSpecification httpRequest = RestAssured.given();
// Make a request to the server by specifying the method Type and the method URL.
// This will return the Response from the server. Store the response in a variable.
Response response = httpRequest.request(Method.GET, "/Hyderabad");
// Now let us print the body of the message to see what response
// we have recieved from the server
String responseBody = response.getBody().asString();
System.out.println("Response Body is => " + responseBody);
}
}
The above code will produce the same response which was received when opened the same URL on a browser. Here is how the response will be printed in Eclipse console window.
Understanding the code
It is very important to understand individual lines of code. Let's start with the first line of code in the test.
Code line 1
// Specify the base URL to the RESTful web service
RestAssured.baseURI = "https://demoqa.com/utilities/weather/city";
This line uses a class called RestAssured to set up a request with the specified base URI. In our case the base URI is "https://restapi.demoqa.com/utilities/weather/city" . This is called the base URI because the is root address of the resource. Adding "/Hyderabad" at the end appends the exact resource name in the URI that we are trying to access.
Coming back to the class io.restassured.RestAssured , this class forms the basis of any kind of HTTP request that is required in the tests. Some key features of this class are
- It creates HTTP Requests against a base URI
- It supports creating Request of different HTTP method types (GET, POST, PUT, PATH, DELETE, UPDATE, HEAD and OPTIONS)
- It makes HTTP communication with the server and passes on the Request that we created in our tests to the server.
- Retrieves the Response from the server.
- Helps validate the Response received from the server.
Internally this class uses HTTP builder library, Http builder is a Groovy language based HTTP client.
Code line 2
Once the request is set up, store the Request in a variable so that it can be modified. In this particular test, it is not required to modify the test. Still following the same approach to understand the basics.
// Get the RequestSpecification of the request that you want to sent
// to the server. The server is specified by the BaseURI that we have
// specified in the above step.
RequestSpecification httpRequest = RestAssured.given();
Here RestAssured class is returning the Request against the base URI, as specified in line 1. Every Request in Rest-Assured library is represented by an interface called RequestSpecification. This interface allows to modify the request, like adding headers or adding authentication details. The word specification at the end is used to signify that how the request should look like, when sent to the server.
Code line 3
Now that RequestSpecification object is there, call the server to get the resource. This piece of code tells RequestSpecification to issue a request to the server.
// Make a request to the server by specifying the method Type and the method URL.
// This will return the Response from the server. Store the response in a variable.
Response response = httpRequest.request(Method.GET, "/Hyderabad");
Issuing request takes two arguments, first argument as HTTP Method Type and second as String ("/Hyderabad"). This step actually sends the request to the remote server and gets a response back. This is why the return type of the request is specified as Response.
In Rest-Assured io.restassured.response.Response interface represents a Response returned from a server. This Response object will contain all the data sent by the server. Different method can be called on the Response object to get different parts of the Response. For e.g. call to get Headers, Status code and the body of the Response. In the next code line we will get the body of the Response.
Code line 4 and 5
This piece of code just tries to read the response and print the response.
// Now let us print the body of the message to see what response
// we have recieved from the server
String responseBody = response.getBody().asString();
System.out.println("Response Body is => " + responseBody);
Response interface has a method called getBody() , this method will return the body of the received response. Response Body is converted into a string value and printed on the console using the System.out.println statement.
Rest-Assured provides a lot of short hand methods which can help you write short code. The above test method can be written in a little different way, here is the code snippet. Please go through the code comments to understand the usage.
@Test
public void GetWeatherDetailsCondensed()
{
// Specify the base URL to the RESTful web service
RestAssured.baseURI = "https://demoqa.com/utilities/weather/city";
// Get the RequestSpecification of the request that you want to sent
// to the server. The server is specified by the BaseURI that we have
// specified in the above step.
RequestSpecification httpRequest = RestAssured.given();
// Make a GET request call directly by using RequestSpecification.get() method.
// Make sure you specify the resource name.
Response response = httpRequest.get("/Hyderabad");
// Response.asString method will directly return the content of the body
// as String.
System.out.println("Response Body is => " + response.asString());
}
The same example will be continued in the Next Chapter to learn more about the usage of io.restassured.response.Response interface.
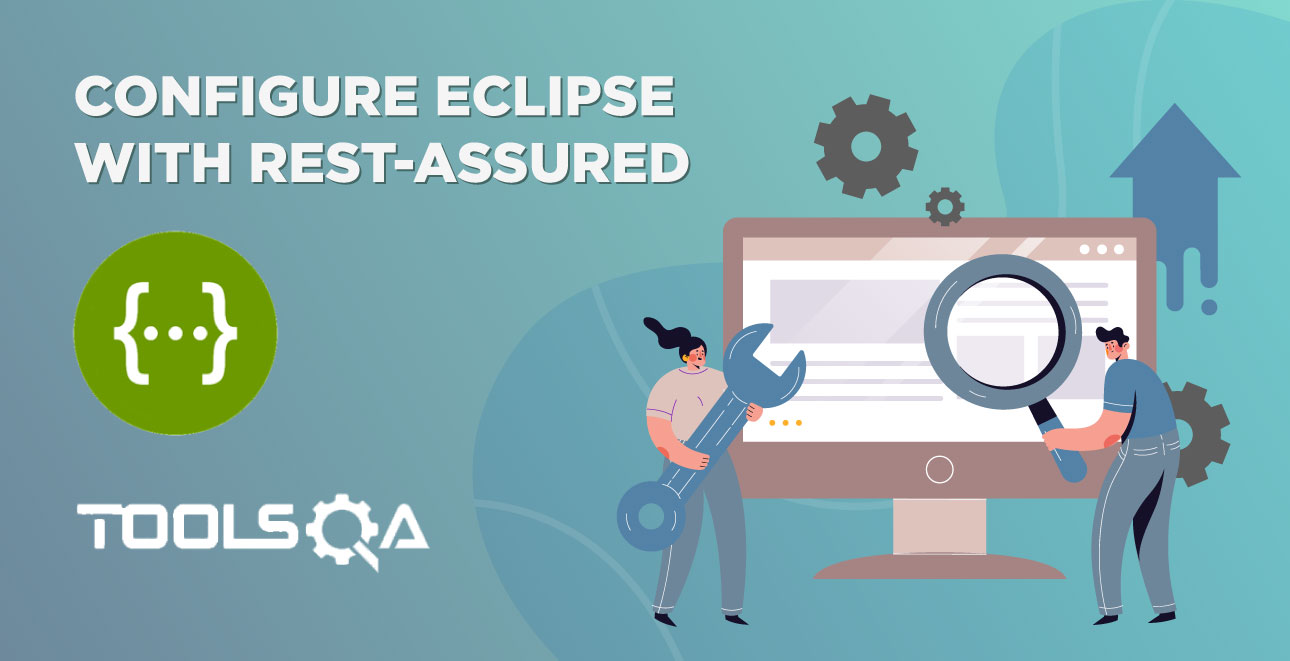
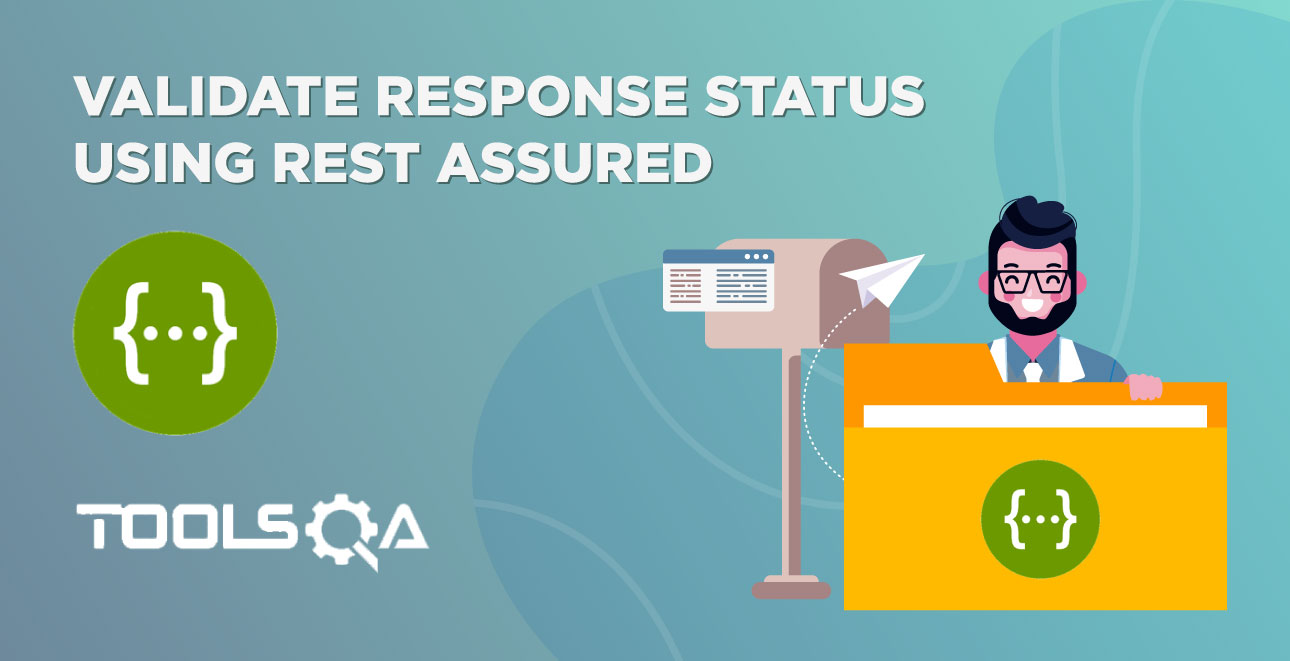