Previous two tutorials covers How to make a simple GET request and How to validate Response Status Code from Weather Web Service. It is suggested to go through the above tutorials first before moving with this tutorial which covers How to Validate Response Header using Rest Assured.
Validate Response Header using Rest Assured
Every response that is received from a server contains zero or more headers. Headers are the part of Response that is sent by the server. Each header entry is basically a Key-Value pair. Headers are used to send across extra information by the server. This extra information is also referred to as Metadata of the Response.
Using the Headers a client can take intelligent decisions for e.g.
One of the Headers called Content-Type which tells how to interpret the data present in the Body of the Response. If the Body contains data in the form of JSON, then the value of Content-Type header will be application/json. Similarly, if the data in the body is XML the Content-Type header will be application/xml.
How to read different Header Types from HTTP Response?
Let's just see how to read a Header using Rest-Assured. To do that let's do a simple exercise in which the test would record the following Header Types from the Response:
- Content-Type
- Server
- Content-Encoding
The Response interface provides direct methods to access individual header or all the Headers. Simply do a Response followed by a dot (Response.head), all the available methods to get headers will be displayed. Below image shows the available methods
In the below code, .header(String arg0) method is used to get a particular header. In the argument of this method pass the exact header name.
@Test
public void GetWeatherHeaders()
{
RestAssured.baseURI = "https://restapi.demoqa.com/utilities/weather/city";
RequestSpecification httpRequest = RestAssured.given();
Response response = httpRequest.get("/Hyderabad");
// Reader header of a give name. In this line we will get
// Header named Content-Type
String contentType = response.header("Content-Type");
System.out.println("Content-Type value: " + contentType);
// Reader header of a give name. In this line we will get
// Header named Server
String serverType = response.header("Server");
System.out.println("Server value: " + serverType);
// Reader header of a give name. In this line we will get
// Header named Content-Encoding
String acceptLanguage = response.header("Content-Encoding");
System.out.println("Content-Encoding: " + acceptLanguage);
}
In this code we are trying to get the Content-Type, Server and Content-Encoding headers. The exact values will be printed out in the Console window using the System.out.println statement. The out of this test looks like this
Note: Response.GetHeader(String headerName) method does exactly the same thing as the Response.Header(String headerName) method does. So the above can be written with replacing .Header with .GetHeader. Try it out yourself.
How to Print all the Headers from HTTP Response?
All the headers in a Response can also be printed by simply iterating over each Header. Response interface provides two methods
- headers() : returns Headers
- getHeaders() : returns Headers
This collection is represented by a class called io.restassured.http.Headers. Headers class implements the Iterable interface. Hence, for each (for( : )) loop can be used to read all the headers, as shown in the code below:
@Test
public void IteratingOverHeaders()
{
RestAssured.baseURI = "https://restapi.demoqa.com/utilities/weather/city";
RequestSpecification httpRequest = RestAssured.given();
Response response = httpRequest.get("/Hyderabad");
// Get all the headers. Return value is of type Headers.
// Headers class implements Iterable interface, hence we
// can apply an advance for loop to go through all Headers
// as shown in the code below
Headers allHeaders = response.headers();
// Iterate over all the Headers
for(Header header : allHeaders)
{
System.out.println("Key: " + header.getName() + " Value: " + header.getValue());
}
}
How to Validate Response Header using Rest Assured?
Now that we have a mechanism to read a Header. Let's write a test to validate the values of the header by putting an Assert. The code is simple and its mostly same as the above code. The only difference is that instead of having a print statement, TestNg Assert is used. Here is the code.
//@Test
public void GetWeatherHeaders()
{
RestAssured.baseURI = "https://restapi.demoqa.com/utilities/weather/city";
RequestSpecification httpRequest = RestAssured.given();
Response response = httpRequest.get("/Hyderabad");
// Reader header of a give name. In this line we will get
// Header named Content-Type
String contentType = response.header("Content-Type");
Assert.assertEquals(contentType /* actual value */, "application/json" /* expected value */);
// Reader header of a give name. In this line we will get
// Header named Server
String serverType = response.header("Server");
Assert.assertEquals(serverType /* actual value */, "nginx/1.12.1" /* expected value */);
// Reader header of a give name. In this line we will get
// Header named Content-Encoding
String contentEncoding = response.header("Content-Encoding");
Assert.assertEquals(contentEncoding /* actual value */, "gzip" /* expected value */);
}
The next tutorial is about Reading and Validating Response Body.
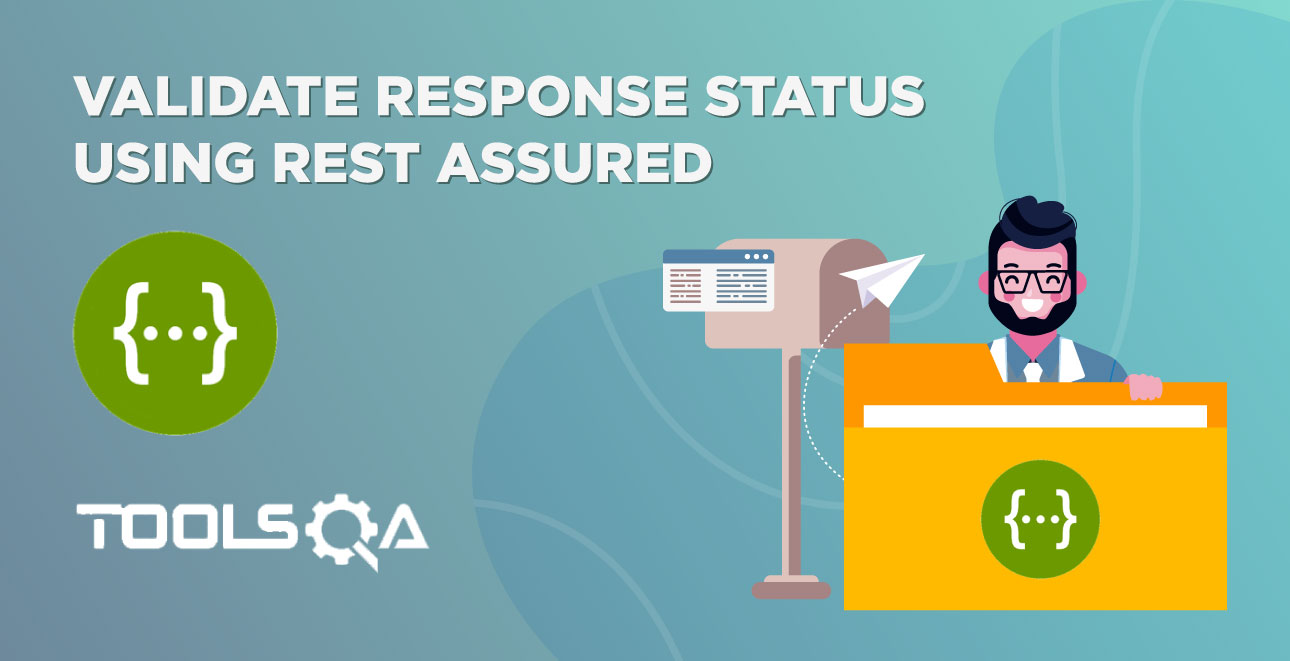
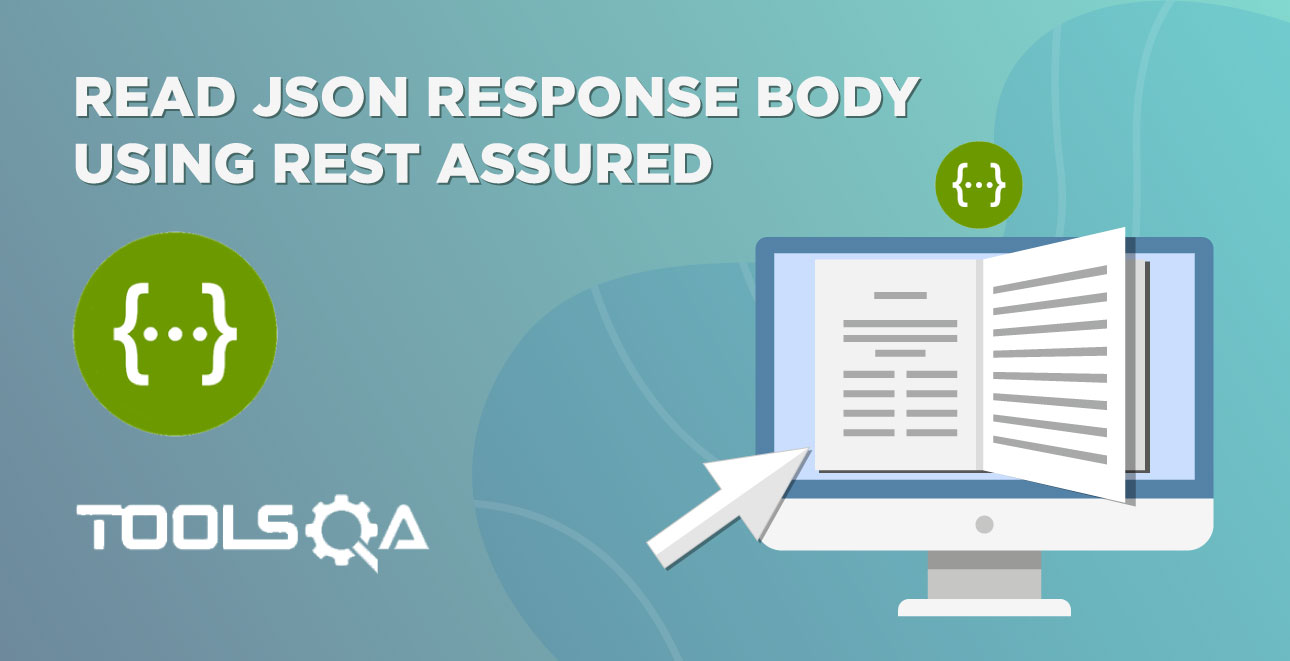