In the previous tutorial of Rest-Assured Test, a test made a call to Weather Web Service. This tutorial will also continue the same example.
Functional testing of Web Services involves verifying the Responses returned from various End Points. Some of the important Test Verification are:
- Response Status
- Response Header
- Response Body
Validate Response Status using Rest Assured
This tutorial will cover the following verification :
- Validating Response Status Code
- Validating Error Status Code
- Validating Response Status Line
In the next tutorial will cover the verification of Response Body Content.
From the previous First Rest-Assured Test example, a simple test which calls the Weather web service using a Get Method looks like this
@Test
public void GetWeatherDetails()
{
// Specify the base URL to the RESTful web service
RestAssured.baseURI = "https://restapi.demoqa.com/utilities/weather/city";
// Get the RequestSpecification of the request that you want to sent
// to the server. The server is specified by the BaseURI that we have
// specified in the above step.
RequestSpecification httpRequest = RestAssured.given();
// Make a GET request call directly by using RequestSpecification.get() method.
// Make sure you specify the resource name.
Response response = httpRequest.get("/Hyderabad");
// Response.asString method will directly return the content of the body
// as String.
System.out.println("Response Body is => " + response.asString());
}
Response object which represents the HTTP Response packet received from the Web service Server. HTTP Response contains Status, collection of Headers and a Body. Hence, it becomes obvious that the Response object should provide mechanisms to read Status, Headers, and Body.
How to Validate Response Status Code?
Response is an interface which lives in the package: io.restassured.response. This interface has lots of methods, mostly methods which can help to get parts of the received response. Take a look at some of the important methods. A simple Response followed by a dot (Response). in eclipse would shown the available methods on the interface. As shown in the image below
Noticed, getStatusCode() method can be used to get the status code of the Response. This method returns an integer and test will verify the value of this integer. TestNG Assert is used to verify the Status Code.
@Test
public void GetWeatherDetails()
{
RestAssured.baseURI = "https://restapi.demoqa.com/utilities/weather/city";
RequestSpecification httpRequest = RestAssured.given();
Response response = httpRequest.get("/Hyderabad");
// Get the status code from the Response. In case of
// a successfull interaction with the web service, we
// should get a status code of 200.
int statusCode = response.getStatusCode();
// Assert that correct status code is returned.
Assert.assertEquals(statusCode /*actual value*/, 200 /*expected value*/, "Correct status code returned");
}
Below line of code extracts the status code from the message:
int statusCode = response.getStatusCode();
Once the status code is received, it is compared with the expected value of 200.
// Assert that correct status code is returned.
Assert.assertEquals(statusCode /*actual value*/, 200 /*expected value*/, "Correct status code returned");
Try running the test and notice that the test will pass. This is because the web service indeed returns a status code of 200. Try to print the value of statusCode.
How to validate an Error Status Code?
Status codes returned by the Server depends on whether the Request was successful or not. If the Request is successful, Status Code 200 is returned. status code. If the Request is not successful, Status Code other than 200 will be returned. Get a list of HTTP Status codes and there meanings on the W3 page.
For ToolsQA Weather web service, let's create another test which tests a negative scenario. The scenario is
- Verify the Status Code returned by Weather web service on providing invalid City name.
City name that this test will use here is a long number, like 78789798798. Just copy the code from previous test and simple replace the city name with 78789798798. At this moment do not change the status code. The code looks like this
@Test
public void GetWeatherDetailsInvalidCity()
{
RestAssured.baseURI = "https://restapi.demoqa.com/utilities/weather/city";
RequestSpecification httpRequest = RestAssured.given();
Response response = httpRequest.get("/78789798798");
int statusCode = response.getStatusCode();
Assert.assertEquals(statusCode /*actual value*/, 200 /*expected value*/, "Correct status code returned");
}
Now run your test class and check the result. Our negative test GetWeatherDetailsInvalidCity will fail. The reason is that the web service returns an error code of 400 when invalid city name is sent to it. However, the test is validating for expected value of 200. Look at the TesNG results, as shown in the image below.
A quick change in the expected result in the code will make sure that this test passes. Update respective line as in the code below
Assert.assertEquals(statusCode /*actual value*/, 400 /*expected value*/, "Correct status code returned");
How to Validate Response Status Line?
The first line returned in the Response from Server is called Status Line. Status line is composed of three sub strings
- Http protocol version
- Status Code
- Status Code's string value
During a success scenario a status line will look something like this "HTTP/1.1 200 OK". First part is Http protocol (HTTP/1.1). Second is Status Code (200). Third is the Status message (OK).
Just as we go the Status Code in the line above, we can get the Status Line as well. To get the Status line we will simply call the Response.getStatusLine() method. Here is the code to do that.
@Test
public void GetWeatherStatusLine()
{
RestAssured.baseURI = "https://restapi.demoqa.com/utilities/weather/city";
RequestSpecification httpRequest = RestAssured.given();
Response response = httpRequest.get("/Hyderabad");
// Get the status line from the Response and store it in a variable called statusLine
String statusLine = response.getStatusLine();
Assert.assertEquals(statusLine /*actual value*/, "HTTP/1.1 200 OK" /*expected value*/, "Correct status code returned");
}
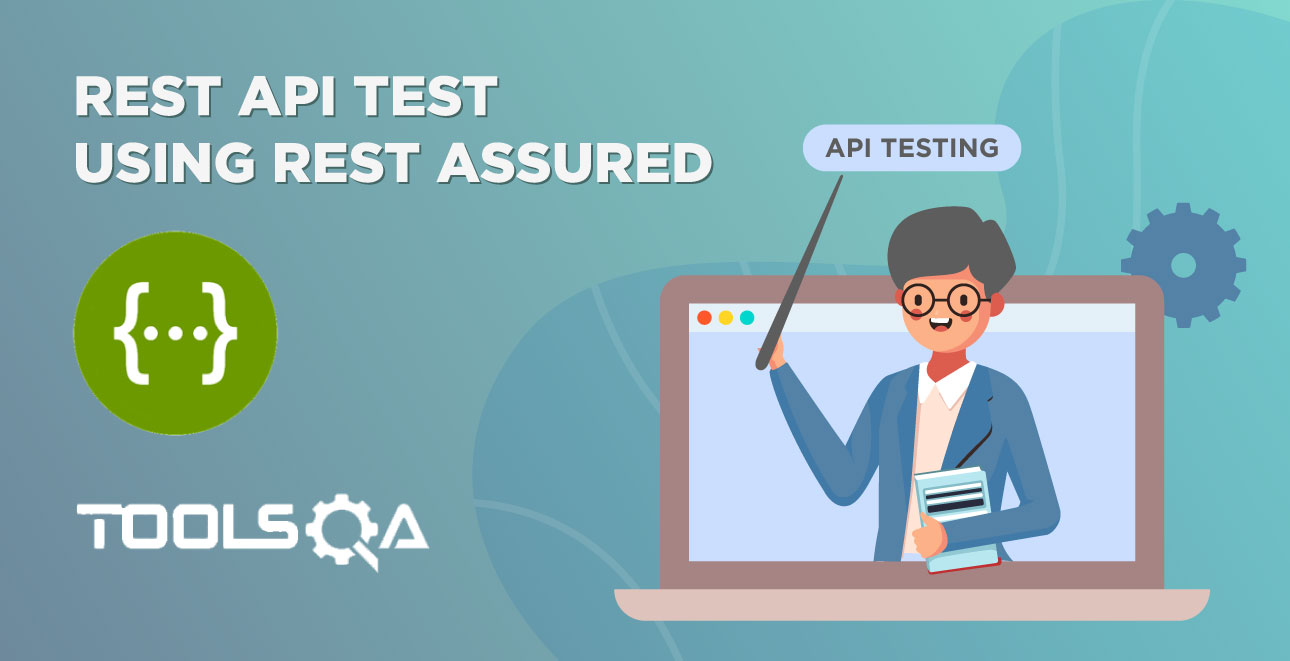
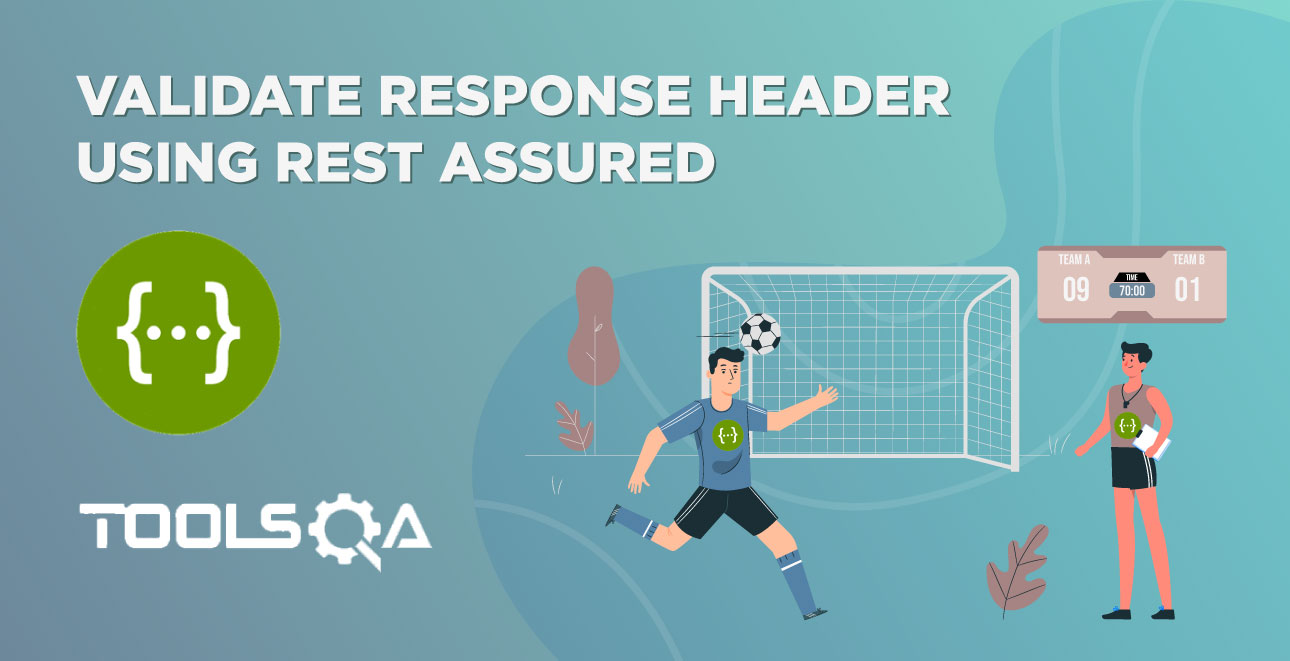