A cheat sheet by name looks like something which is not allowed or which can be misused. In software reference a cheat sheet is something which is used for quick reference or which is used for knowing something too specific without any details mainly codes, syntax or formulas. So this tutorial is designed for your quick reference for some Postman commands, codes and syntax. Please Note that all the syntax and code used here is as of latest style of writing codes/tests in Postman.
For more information you can visit https://learning.getpostman.com/docs/postman/scripts/test_examples/
Variables
Codes for working with environment and variables
Setting Variables
Codes for working around with variables
Global Variables
pm.globals.set('variable name', "value");
Local Variable
var variable_name = value;
Environment Variable
pm.environment.set('variable_name' , 'value');
Getting Variables
Global Variables
pm.globals.get('variable_name');
Environment Variable
pm.environment.get('varibable_name');
Data Variable
pm.iterationData.get('variable_name');
Local Variable
variable_name;
Clearing Variables
Global Variables (only one)
pm.globals.unset('variable_name');
Global Variables (All)
pm.globals.clear();
Environment Variable(only one)
pm.environment.unset('variable_name');
Environment Variable (All)
pm.environment.clear();
Assertions
Response
Different codes related to the response.
Response contains a string
pm.test("String found", function(){
pm.expect(pm.response.text()).to.include("string you want to search");
});
Response body is equal to a string
pm.test("Body is equal to string", function(){
pm.response.to.have.body("string you want to check");
});
Status Code
Code related to the status code in Postman
Single Status code
pm.response.to.have.status(status_code);
Multiple Status Code
pm.expect(pm.response.code).to.be.oneOf([status_code, status_code]);
Response Time
pm.expect(pm.response.responseTime.to.be.below(time));
Check for JSON Value
pm.test("Your_Test_Name", function(){
var jsonData = pm.response.json();
pm.expect(jsonData.value).to.eql(value);
});
Content-Type Present
pm.test("Your_Test_Name", function(){
pm.response.to.have.header("Content-Type");
});
Header Exists
pm.response.to.have.header('X-Cache');
Cookie Exists
pm.expect(pm.cookies.has('sessionID')).to.be.true;
Body
Code related to body field.
Exact Match
pm.response.to.have.body("OK");
pm.response.to.have.body('{"success"=true}');
Partial Match
pm.expect(pm.response.text()).to.include('ToolsQA');
Send an Asynchronous Request
pm.sendRequest("https://postman-echo.com/get", function(err, response){
console.log(response.json());
});
JSON Response
Parse Body
var jsonData = pm.response.json();
Check the array count in the response
pm.test("ISBN Count", function () {
pm.expect(2).to.eql(pm.response.json().arrayName.length);
});
Check for a particular value inside array
This example is checking a particular ISBN number among all the books received in response and returns true if found.
pm.test("Test Name", function () {
var result;
for (var loop = 0; loop < pm.response.json().arrayName.length; loop++)
{
if (pm.response.json().arrayName[loop].arrayElement=== pm.variables.get("arrayElementValue")){
result=true;
break;
}
}
pm.expect(true).to.eql(result);
});
The above two examples have used Javascript for coding as Postman Sandbox works in javascript. This code is not related to Postman in specific. Please refer to Javascript for the same.
Check the value
pm.expect(jsonData.age).to.eql(value);
pm.expect(jsonData.name).to.eql("string");
Convert XML body to JSON object
var jsonObject = xml2Json(responseBody);
Workflows
Setting the next request
postman.setNextRequest("Request Name");
Stop executing the request
postman.setNextRequest(null);
Chai Assertion Library
Find a number present in an array
pm.test(“Number included”, function(){
pm.expect([1,2,3]).to.include(3);
});
Check if an array is empty
pm.test(“Empty Array”, function(){
pm.expect([2]).to.be.an(‘array’).that.is.empty;
});
I hope this will serve as a quick reference point in your postman journey.
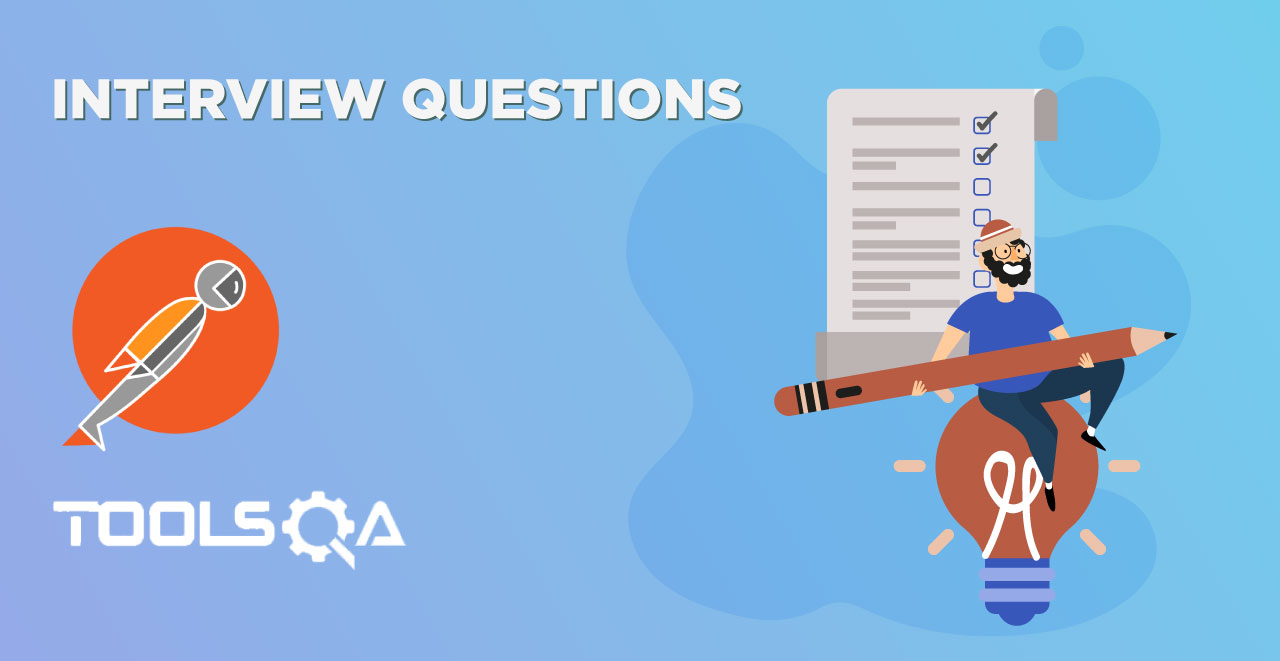