Table of Contents
Selenium Tutorial - WebDriver Basics
Basic Java
- Data Types and Variables
- Operators
- Decision Making
- Arrays
- Loops
- Classes and Objects
- Class Constructors
- String Class
Set Up Selenium WebDriver - Selenium Tutorial
- Set Up Java
- Set Up Eclipse
- Download Selenium WebDriver
- Configure Selenium WebDriver with Eclipse
- First Selenium Test Case
- How to use GeckoDriver in Selenium?
- Running Test on Safari Browser
- Run Selenium tests on Chrome
- Run Selenium Tests on Internet Explorer
- Run Selenium tests on Edge
- Internet Explorer Driver Server
- Challenges to run Selenium Scripts with IE Browser
WebDriver Commands
- Browser Commands
- Navigation Commands
- WebElement Commands
- Find Element and Find Elements in Selenium
- Handle CheckBox in Selenium WebDriver
- Handle Radio Button in Selenium WebDriver
- Handle Dropdown in Selenium
- Handle Dynamic WebTables in Selenium Webdriver
Locators & XPath
- Selenium Locators
- Inspect Elements using Web Inspector
- Inspect Element In Chrome
- XPath, FireBug & FirePath
- XPath in Selenium
- Write Effective XPaths
- CSS Selectors in Selenium
- WebDriver Element Locator Firefox Add On
- XPath Helper
Selenium Tutorial - WebDriver Intermediate
Java Advance
- Modifiers – Access Modifiers
- Modifiers – Non Access Modifiers
- Inheritance
- Polymorphism
- Exception Handling
Switches Alerts & Windows
- Wait Commands
- Advance Webdriver Waits
- Handle Ajax Wait Using JavaScriptExecutor in Selenium?
- Window Handle in Selenium
- PopUps and Alerts in Selenium
- Handling Iframes using Selenium WebDriver
- iFrames in Selenium WebDriver
- @CacheLookup in PageObjectModel
- Handle Unexpected Alert - - Coming Soon
- Expected Condition Wait - - Coming Soon
Action Class
- Actions Class in Selenium
- Right Click and Double Click in Selenium
- Drag and Drop in Selenium
- Mouse Hover action in Selenium
- ToolTip in Selenium
- Keyboard Events in Selenium Actions Class
Robot Class
Tips & Tricks
- Selenium Headless Browser Testing
- Use of AutoIt in Selenium Webdriver
- Handle SSL Certificate in Selenium
- HTTP Proxy Authentication with Selenium Webdriver
- Find Broken Links in Selenium
- Refresh Browser in Different Ways
- Junit Test with Selenium WebDriver
- Upload & Download files - Coming Soon
- Selenium of Exceptions and handling - Coming Soon
Selenium Tutorial - WebDriver Advance
Log4j Logging and it's advance Usage
- Log4j Introduction
- Download Log4j
- Add Log4j Jars
- Test Case with Log4j Logging
- Log4j Manager
- Loggers
- Appenders
TestNG Framework
- TestNG Introduction
- Install TestNG
- TestNG Test
- TestNG Test Suite
- TestNG Annotations
- TestNG Groups
- TestNG Dependent Tests
- TestNG Reports
- TestNG Parameters
- TestNG Data-Providers
- TestNG Test Priority
- TestNG Reporter Log
- TestNG Asserts
- TestNG Cross Browser Testing
- TestNG Data Provider with Excel
- TestNG Parallel Execution
- TestNG Listeners
- Retry Failed Tests in TestNG
- Implement IRetryAnalyzer to Retry Failed Test in TestNG Framework
- TestNG Vs JUnit
- TestNG Interview Questions
DataBase Connections
- Database connection- Coming Soon
- Database Testing in Selenium using MSAccess and Oracle - Coming Soon
Tips & Tricks
- Capturing ScreenShot in Selenium
- WebDriverManager
- Finding broken links in Selenium automation
- Testing Flash with Selenium (Flash – JavaScript communication)
- Custom Firefox Profile for Selenium
- JavaScript and Selenium JavaScriptExecutor
- Scroll Web elements and Web page- Selenium WebDriver using Javascript
- Selenium WebDriver Event Listener
- @CacheLookup in PageObjectModel
Selenium Grid
Interview Questions
Selenium Tutorial - Automation Framework
Design Patterns
Data-Driven Framework
- Apache POI – Download and Installation
- Read & Write Data from Excel in Selenium: Apache POI
- Data-Driven Framework (Apache POI – Excel)
Hybrid Automation Framework
- Automation Framework
- Page Object Model
- Modular Driven Framework
- Functions Parameters
- Constant Variables
- Data-Driven Framework
- Log4j Logging
- TestNG Reporting
- User-Defined Function
- Object Repository
- Exception Handling
- Hybrid Automation Framework
Keyword Driven Framework
- Keyword Driven Framework – Introduction
- Steps to Set up Keyword Driven Framework
- Identify and Implement Action Keywords
- Set Up Data Engine – Apache POI (Excel)
- Use of Java Reflection Class
- Set Up Java Constant Variables
- Object Repository
- Test Suite Execution Engine
- Log4j Logging
- Exception Handling
- Test Result Reporting
- Data-Driven Technique
- Framework for Manual Testers
- Project Code Base
Framework Designing Principles
- Factory design principle in Selenium Tests
- Object Repository using a Properties file
- Object Repository for Selenium using JSON
- Page Factory in Selenium
- Hard Asserts & Soft Asserts - Coming Soon
Continuous Integration
Maven
- Maven Introduction
- Install Maven in Eclipse IDE
- Install Maven on Windows
- Install Maven on Mac
- How to Create a New Maven Project
- How to Create a New Maven Project in Eclipse
- Send Reports Automatically to Email using Maven from Eclipse
Jenkins
- Jenkins Overview
- Install Jenkins
- How to install Tomcat and deploy Jenkins on it?
- Jenkins GitHub Integration
- Jenkins Maven Configuration
- Jenkins Configuration
- Manage Jenkins
- Jenkins Build Jobs
- Jenkins User Management
- Jenkins Reports
- Jenkins Distributed Builds
- Jenkins Automated Deployment
- Jenkins – Metrics and Trends
- Jenkins Manage Plugins
- Jenkins Pipeline
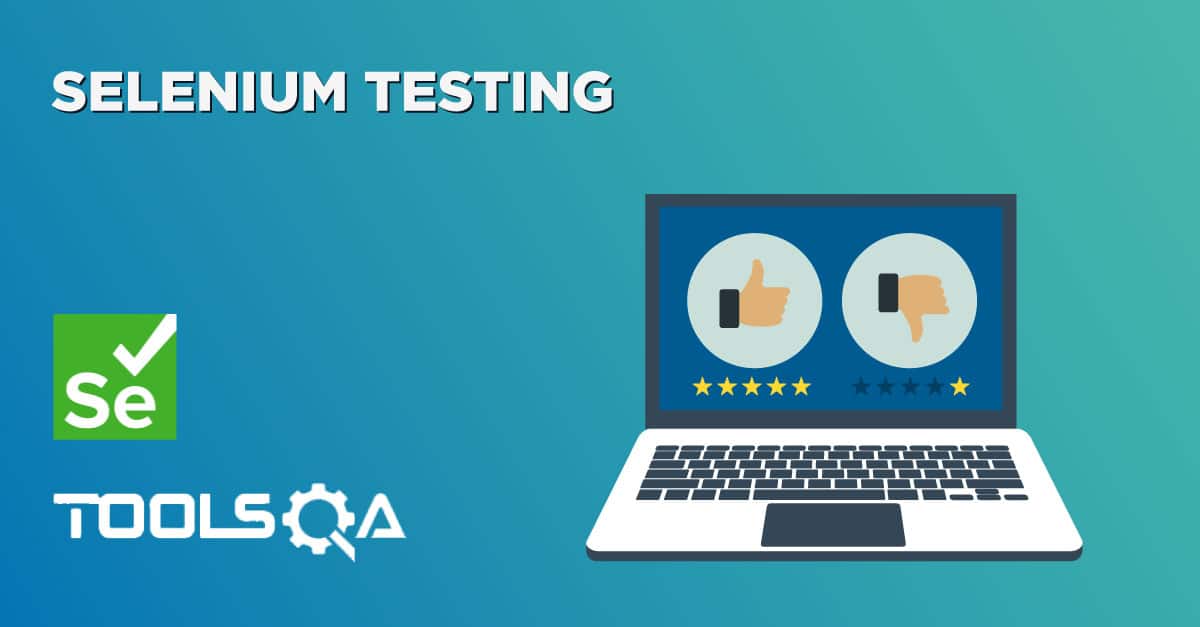